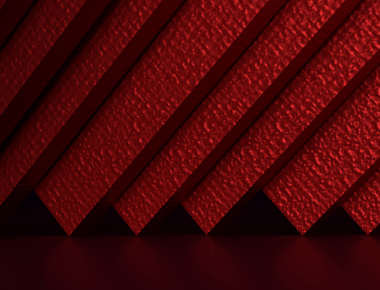
Angular Supports two ways of building forms. One is a template-driven approach, the other is called reactive form. Here, we will learn how to create a simple Template-driven forms example app. We first build a simple HTML form with just a few form elements. The ngForm
directive will convert it into a Template-driven form and create the top-level FormGroup
control. Next, we’ll use the ngModel
directive to create FormControl
objects for each of the HTML form elements. Later, we will learn about how to submit the form data to the component class and also learn how to initialize or reset form data and how to use the data binding features.
Template-driven forms require directives and attributes to create predefined behaviors/validations in our template and let it work in the background. All things happen in Templates, so very little code is needed in the component class. This is different from the reactive forms. In those, we define the logic and controls in the component class.
import { FormsModule } from '@angular/forms' @NgModule({ imports: [FormsModule] //... }) export class AppModule {}
<form #myForm="ngForm" (ngSubmit)="onSubmit(myForm)"> <div> <label for="username">Username:</label> <input type="text" id="username" name="username" [(ngModel)]="user.username" required> <div *ngIf="username.invalid && username.touched">Username is required.</div> </div> <div> <label for="email">Email:</label> <input type="email" id="email" name="email" [(ngModel)]="user.email" required email> <div *ngIf="email.invalid && email.touched">Please enter a valid email.</div> </div> <button type="submit" [disabled]="myForm.invalid">Submit</button> </form>
import { Component } from '@angular/core' @Component({ selector: 'app-template-form', templateUrl: './template-form.component.html' }) export class TemplateFormComponent { user = { username: '', email: '' } onSubmit(form) { console.log('Form submitted:', form.value) } }
In the template above, the form model (myForm) is automatically created by Angular and bound to the form tag using the #myForm=“ngForm” local template variable. When the form is submitted, the onSubmit method is called, and the form value is printed to the console.
Template-driven forms in Angular provide a declarative way to manage form data, allowing developers to leverage the power of the framework’s directives to handle form validation, data-binding, and submission with minimal logic required in the component class. By utilizing the ’FormsModule’, ’ngModel’, and other directives, Angular empowers developers to create robust forms directly within templates. This approach simplifies the development process for scenarios where form requirements are straightforward and where most of the logic can be handled at the template level. In the provided example, it becomes evident how seamless the integration between the HTML form and the component class is, making form management in Angular efficient and intuitive. Whether you’re just starting out or a seasoned developer, understanding template-driven forms is a foundational skill for developing interactive applications in Angular.
Quick Links
Legal Stuff