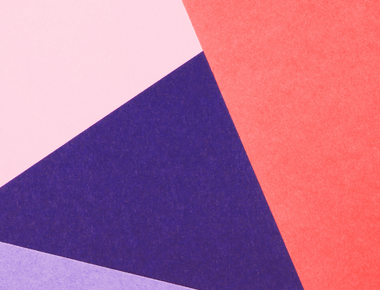
Modern web applications often require the capability to push real-time updates to connected clients, be it a chat application, stock ticker, or live game score updates. Enter SignalR – a library in the ASP.NET Core ecosystem that provides real-time web functionality, enabling server-side code to push content to connected clients instantly.
a. Create a new ASP.NET Core Web App:
dotnet new webapp -n SignalRChat
b. Add the SignalR package:
dotnet add package Microsoft.AspNetCore.SignalR
Hubs provide a high-level API for sending messages to clients. Let’s set up a chat hub:
using Microsoft.AspNetCore.SignalR; public class ChatHub : Hub { public async Task SendMessage(string user, string message) { await Clients.All.SendAsync("ReceiveMessage", user, message); } }
a. In ’ConfigureServices‘:
public void ConfigureServices(IServiceCollection services) { services.AddSignalR(); // other code... }
b. In ’Configure‘:
public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { // other code... app.UseEndpoints(endpoints => { endpoints.MapHub<ChatHub>("/chathub"); }); }
Assuming you’re using the default template, open ’Pages/Index.cshtml‘:
a. Add references for SignalR:
<script src='https://cdnjs.cloudflare.com/ajax/libs/microsoft-signalr/3.1.9/signalr.min.js'></script>
b. Implement the chat logic:
let connection = new signalR.HubConnectionBuilder().withUrl('/chathub').build() connection.on('ReceiveMessage', function (user, message) { let encodedMessage = `${user}: ${message}` let listItem = document.createElement('li') listItem.textContent = encodedMessage document.getElementById('messagesList').appendChild(listItem) }) connection.start().catch(function (err) { return console.error(err.toString()) }) document .getElementById('sendButton') .addEventListener('click', function (event) { let user = document.getElementById('userInput').value let message = document.getElementById('messageInput').value connection.invoke('SendMessage', user, message).catch(function (err) { return console.error(err.toString()) }) event.preventDefault() })
c. Add the necessary HTML (a simple form and a list):
<input type="text" id="userInput" /> <input type="text" id="messageInput" /> <button id="sendButton">Send</button> <ul id="messagesList"></ul>
dotnet run
Visit the web page, enter a username and message, and see the real-time chat in action!
SignalR offers a powerful and efficient means to introduce real-time functionality into web applications. With its automatic connection management, robust fallback mechanisms, and inherent scalability, it makes the implementation of dynamic features like live chat applications straightforward. As demonstrated with the basic chat application setup, integrating SignalR into an ASP.NET Core project is relatively seamless. By utilizing SignalR, developers can provide a more interactive and responsive user experience, further bridging the gap between traditional web applications and real-time systems. Whether you’re aiming to deliver instant notifications, live game scores, or real-time collaborative tools, SignalR stands as a pivotal tool in the modern web developer’s toolkit.
Quick Links
Legal Stuff