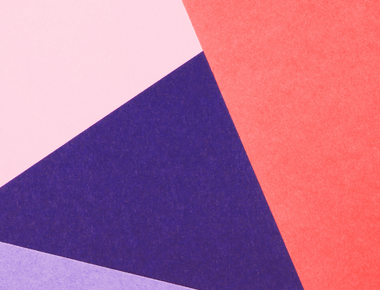
The ever-evolving world of React has brought forth another fascinating development: React Server Components. With performance and scalability at the heart of modern web apps, the React team introduces a feature that could redefine how we architect and deliver our applications. This blog post aims to dive into React Server Components, helping you grasp their essence and potential.
React Server Components (RSC) are a new type of component introduced by the React team that render exclusively on the server. Unlike traditional React components that execute in the browser, RSC run on the server, sending the resultant HTML and minimal JavaScript to the client. This process reduces the client-side workload, potentially leading to faster load times and a more interactive experience.
The server renders the components and sends back a special format to the client. This format can be deeply nested, allowing for compositions of server and client components. When the data changes, only the affected server components re-render, and the minimal diffs are sent to the client to be applied.
Here’s a basic example to demonstrate React Server Components:
// UserProfile.server.js import { db } from './database' // Some hypothetical database utility function UserProfile({ userId }) { // Fetch user data directly from the database since we are on the server const user = db.getUserById(userId) return ( <div> <h1>{user.name}</h1> <p>{user.description}</p> </div> ) } export default UserProfile
// App.js import React from 'react' import UserProfile from './UserProfile.server' // Importing the server component function App() { return ( <div> <h1>Welcome to React Server Components</h1> <UserProfile userId={1} /> </div> ) } export default App
While a detailed guide would be expansive, here’s a basic setup:
React Server Components represent a transformative step in the evolution of React, offering a paradigm shift that emphasizes performance, efficiency, and cleaner code. By rendering components on the server and sending minimal HTML and JavaScript to the client, developers can achieve faster load times and improved user interactivity without compromising on the rich features and interactivity that React offers. With direct backend access, reduced client-side fetching logic, and inherent performance optimizations, RSC is poised to redefine web app architecture. As with any new technology, it’s crucial to familiarize oneself with its nuances and potential. React Server Components present an exciting opportunity for developers to create more efficient, scalable, and user-friendly applications, marking another milestone in the continuous journey of web development excellence.
Quick Links
Legal Stuff