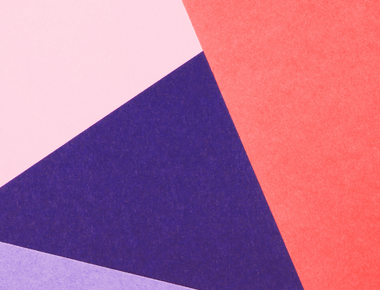
React Native has rapidly grown into one of the most popular frameworks for building mobile applications. One of the reasons behind its widespread adoption is the rich ecosystem of libraries and tools that augment and simplify the development process. In this blog post, we’ll explore some of the most essential and trending React Native libraries and tools that every developer should be aware of.
Navigating between screens is fundamental in mobile apps. React Navigation is a powerful library that offers stack, tab, drawer, and other types of navigational patterns. Its flexibility, active community support, and constant updates make it a go-to choice.
import { NavigationContainer } from '@react-navigation/native' import { createStackNavigator } from '@react-navigation/stack' const Stack = createStackNavigator() function App() { return ( <NavigationContainer> <Stack.Navigator> <Stack.Screen name='Home' component={HomeScreen} /> <Stack.Screen name='Details' component={DetailsScreen} /> </Stack.Navigator> </NavigationContainer> ) }
Managing state in larger apps can become convoluted. Redux, combined with React-Redux, offers a predictable state container that integrates well with React Native apps.
import { createStore } from 'redux' import { Provider } from 'react-redux' const initialState = { count: 0 } const reducer = (state = initialState, action) => { switch (action.type) { case 'INCREMENT': return { count: state.count + 1 } default: return state } } const store = createStore(reducer) function App() { return ( <Provider store={store}> <Counter /> </Provider> ) }
For a consistent and customizable UI, NativeBase provides a suite of components that are both elegant and functional.
Example:
First, you need to install the library:
npm install native-base
Here’s a simple example of a React Native app using NativeBase components:
import React from 'react' import { SafeAreaView, StatusBar } from 'react-native' import { Container, Header, Title, Content, Footer, FooterTab, Button, Left, Right, Body, Icon, Text } from 'native-base' function App() { return ( <SafeAreaView style={{ flex: 1 }}> <StatusBar barStyle='light-content' /> <Container> <Header> <Left> <Button transparent> <Icon name='menu' /> </Button> </Left> <Body> <Title>NativeBase App</Title> </Body> <Right /> </Header> <Content padder> <Button full primary> <Text>Click Me</Text> </Button> </Content> <Footer> <FooterTab> <Button vertical> <Icon name='apps' /> <Text>Apps</Text> </Button> <Button vertical> <Icon name='camera' /> <Text>Camera</Text> </Button> <Button vertical active> <Icon active name='navigate' /> <Text>Navigate</Text> </Button> <Button vertical> <Icon name='person' /> <Text>Contact</Text> </Button> </FooterTab> </Footer> </Container> </SafeAreaView> ) } export default App
Styled Components bring the power of CSS-in-JS to React Native. It helps in writing clean and reusable components.
import styled from 'styled-components/native' const StyledButton = styled.TouchableOpacity` padding: 10px; background-color: blue; ` function App() { return <StyledButton onPress={() => alert('Pressed!')} /> }
Facebook’s Flipper is a comprehensive debugging tool that offers a variety of plugins for inspecting, tracking, and debugging React Native apps.
Lottie by Airbnb offers a way to bring high-quality animations into your React Native app. Import animations created in tools like After Effects directly into your project.
Formik takes the pain out of forms in React Native. It simplifies form management, validation, and error handling.
Here’s a brief overview of using Formik with React Native:
npm install formik
Let’s create a simple login form using Formik:
import React from 'react' import { TextInput, Button, Text, View } from 'react-native' import { Formik } from 'formik' function LoginForm() { return ( <Formik initialValues={{ email: '', password: '' }} onSubmit={values => console.log(values)} validate={values => { const errors = {} if (!values.email) { errors.email = 'Email is required' } if (!values.password) { errors.password = 'Password is required' } return errors }} > {({ handleChange, handleBlur, handleSubmit, values, errors }) => ( <View> <TextInput placeholder='Email' onChangeText={handleChange('email')} onBlur={handleBlur('email')} value={values.email} /> {errors.email && ( <Text style={{ color: 'red' }}>{errors.email}</Text> )} <TextInput placeholder='Password' onChangeText={handleChange('password')} onBlur={handleBlur('password')} value={values.password} secureTextEntry /> {errors.password && ( <Text style={{ color: 'red' }}>{errors.password}</Text> )} <Button onPress={handleSubmit} title='Submit' /> </View> )} </Formik> ) } export default LoginForm
In this example:
For more complex validation requirements, Yup is commonly used with Formik. Yup provides a way to create validation schemas which Formik can utilize.
npm install yup
import * as Yup from 'yup' const validationSchema = Yup.object().shape({ email: Yup.string().email('Invalid email').required('Email is required'), password: Yup.string() .min(5, 'Password must be at least 5 characters') .required('Password is required') }) // In your Formik component: ;<Formik initialValues={{ email: '', password: '' }} onSubmit={values => console.log(values)} validationSchema={validationSchema} > {/* ... */} </Formik>
For basic, unencrypted, persistent local storage, AsyncStorage is a simple key-value storage system that’s built into React Native.
React Native has indeed positioned itself as a frontrunner in mobile application development, largely due to its expansive ecosystem of libraries and tools that cater to a plethora of development needs. From seamless navigation with React Navigation to the elegant UI elements of NativeBase, from the robust state management capabilities of Redux to the dynamic animations made possible by Lottie, developers are equipped with a vast array of resources. Furthermore, with utilities like Formik and Yup, form management and validation become streamlined, while debugging is made hassle-free with tools like Flipper. And, for those crucial storage needs, AsyncStorage offers an in-built solution. In sum, the evolving landscape of React Native development is not just about building mobile applications; it’s about building efficient, beautiful, and high-performance applications with ease. Whether you’re a newbie or a seasoned developer in the React Native world, these tools and libraries are instrumental in enhancing productivity and refining the overall development experience.
Quick Links
Legal Stuff