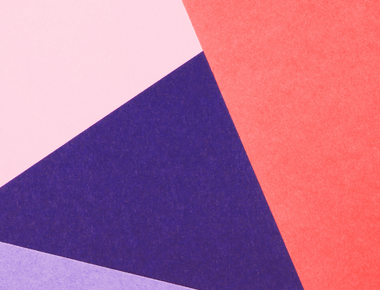
The React ecosystem is continually evolving, and with the introduction of Concurrent Mode, the React team has once again showcased its commitment to improving user interface experiences. This post aims to demystify Concurrent Mode, guiding you through its nuances and showing how it can revolutionize the way we write React apps.
Concurrent Mode is an advanced set of features in React that helps apps remain responsive while rendering complex UIs. It lets React interrupt a long-running render to handle tasks like user interactions, keeping your app smooth and snappy.
In essence, Concurrent Mode allows multiple tasks to be processed in the background, interweaving them, so the main thread isn’t blocked.
Think about those times when your React app takes a little too long to update after a user action, or when animations stutter. This lag can lead to a poor user experience. With Concurrent Mode, React can pause ongoing tasks, attend to more urgent ones, and then resume the paused tasks, ensuring that users get a smooth experience.
To opt into Concurrent Mode, you would wrap your app or part of your app with a ’ConcurrentMode’ wrapper:
import React from 'react' import ReactDOM from 'react-dom' ReactDOM.createRoot(document.getElementById('root')).render(<App />)
Note: Concurrent Mode is an opt-in feature, meaning you have to deliberately choose to use it. Not all React features are compatible with it out of the box, so it’s crucial to test thoroughly when enabling it.
Let’s dive into an example using Suspense for data fetching:
import React, { Suspense } from 'react' // A mock API to simulate data fetching function fetchUserData(userId) { return new Promise(resolve => { setTimeout(() => { switch (userId) { case '1': resolve({ name: 'John Doe' }) break case '2': resolve({ name: 'Jane Doe' }) break default: throw new Error('Unknown user.') } }, 2000) }) } const resource = fetchUserData('1') function UserProfile() { const user = resource.read() // This would actually be more complex in a real use-case return <h1>{user.name}</h1> } function App() { return ( <Suspense fallback={<h1>Loading...</h1>}> <UserProfile /> </Suspense> ) } export default App
React’s Concurrent Mode introduces a groundbreaking paradigm to optimize and prioritize tasks, ensuring that applications remain responsive even under heavy rendering loads. By providing mechanisms like interruptible rendering, improved data fetching with Suspense, and automatic prioritization, Concurrent Mode holds the promise of redefining the way developers approach UI responsiveness in React applications. However, as with all powerful tools, it comes with its nuances. Developers must be cautious about compatibility issues and are strongly encouraged to use features like Error Boundaries for graceful error handling. As always, rigorous testing remains paramount, especially when integrating Concurrent Mode into existing applications. Embracing these best practices will enable developers to leverage the full potential of Concurrent Mode, delivering smoother and more interactive user experiences.
Quick Links
Legal Stuff