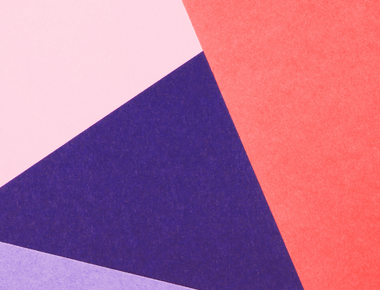
Python, with its clear syntax, vast libraries, and active community, has become a favorite among developers. But sometimes, to leverage a particular functionality or enhance performance, there’s a need to integrate Python with other languages. Thankfully, the Python ecosystem is rich with tools and strategies to make this integration seamless. Let’s delve into the fascinating world of blending Python with other programming languages.
There are several compelling reasons to combine Python with other languages:
C and C++ with Python
Java with Python
.NET with Python
Rust with Python
Here are a few ways you can integrate Python with other languages:
Python provides a way to write modules in C that can be imported into Python programs. This can be useful for optimizing performance-critical sections of your code.
Example - A simple C extension for Python that adds two numbers.
#include <Python.h> // Function to add two numbers static PyObject* add(PyObject* self, PyObject* args) { int a, b; if (!PyArg_ParseTuple(args, "ii", &a, &b)) { return NULL; } return PyLong_FromLong(a + b); } // Module's method table static PyMethodDef AddMethods[] = { {"add", add, METH_VARARGS, "Add two numbers"}, {NULL, NULL, 0, NULL} }; // Module definition static struct PyModuleDef addmodule = { PyModuleDef_HEAD_INIT, "add_module", NULL, -1, AddMethods }; // Module initialization PyMODINIT_FUNC PyInit_add_module(void) { return PyModule_Create(&addmodule); }
Compile:
gcc -shared -o add_module.so -fPIC -I/usr/include/python3.x add_module.c
Use in Python:
import add_module result = add_module.add(3, 5) print(result)
Cython is a language that makes it easy to write C extensions for Python. It looks much like Python but also supports calling C functions and declaring C types.
def add(int a, int b): return a + b
Compile:
cython add_module.pyx --embed gcc -o add_module $(python3-config --cflags --ldflags) add_module.c
Both ’ctypes’ and ’cffi’ are Python libraries that allow calling functions in shared libraries/DLLs and defining C types in Python using C syntax.
Example with ’ctypes‘:
from ctypes import cdll # Load shared library lib = cdll.LoadLibrary('./add_module.so') # Call function result = lib.add(3, 5) print(result)
Integrating Python with other programming languages offers an exciting opportunity to harness the strengths of both worlds. Whether aiming for performance improvements with C, C++, or Rust, or leveraging specialized libraries from Java and .NET environments, the Python ecosystem provides a plethora of tools to achieve seamless integration. While these integration strategies can unlock numerous benefits, developers must remain mindful of potential challenges like added complexity, debugging hurdles, and data transfer overhead. By carefully choosing the right integration approach and toolset, teams can craft solutions that are both efficient and maintainable. As we progress in the world of programming, such integrative endeavors will become increasingly important, highlighting the versatility and adaptability of Python in diverse software landscapes.
Quick Links
Legal Stuff