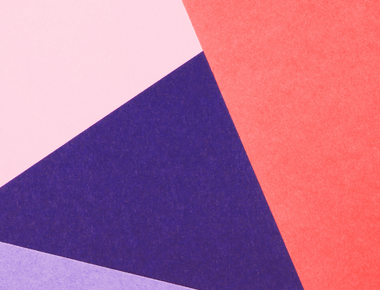
Since their introduction in React 16.8, Hooks have unquestionably altered the landscape of React development. The once dominant class components with their lifecycle methods took a backseat, making way for the rise of more succinct and powerful functional components. In this post, we’ll traverse the journey of Hooks, from their inception to the innovation they’ve driven in modern React applications.
Before diving into Hooks’ evolution, it’s essential to understand the scene before their arrival:
State and lifecycle methods were confined to class components.
import React, { Component } from 'react' class Counter extends Component { constructor(props) { super(props) this.state = { count: 0 } } componentDidMount() { // side effects, like API calls, subscriptions, etc. } increment = () => { this.setState(prevState => ({ count: prevState.count + 1 })) } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={this.increment}>Increment</button> </div> ) } } export default Counter
React 16.8 introduced Hooks as a new way to use state and other React features without writing a class. The most foundational of these were:
The community’s response was overwhelming. Developers appreciated the simplification, and it became evident that Hooks were not just a feature, but a transformative shift.
With the ability to abstract and share component logic, developers began crafting custom Hooks:
Custom Hooks bolstered code reuse and abstraction in applications, leading to cleaner and more maintainable codebases.
React’s core team introduced more specialized Hooks:
Furthermore, the community created libraries like ’react-query’ for server state and data synchronization, ’zustand’ for lean global state management, and many more.
With React 16.8, Hooks like useState and useEffect were introduced, allowing functional components to have state and side effects.
import React, { useState, useEffect } from 'react' function Counter() { const [count, setCount] = useState(0) useEffect(() => { // side effects, equivalent to componentDidMount and componentDidUpdate combined // for example, an API call, subscription, etc. return () => { // cleanup, equivalent to componentWillUnmount // for example, canceling subscriptions, timers, etc. } }, [count]) // The effect will re-run if count changes return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ) } export default Counter
While Hooks brought significant advantages, they weren’t without criticisms:
React’s direction is evident: Hooks are here to stay. With continuous community contributions and potential innovations from the React core team, we can anticipate further evolution and refinement of the Hooks API.
React’s introduction of Hooks marked a pivotal shift in the way developers approach state management and side effects in their applications. Trading the verbosity of class components for the elegance and power of functional components, Hooks have paved the way for a more streamlined, efficient, and modular React development experience. While they’re not without challenges, their immense impact on the ecosystem, from simplifying the learning curve to spurring innovations in third-party libraries, is undeniable. As we look ahead, with the unwavering support from the community and the proactive stance of the React core team, Hooks promise a dynamic and continually evolving future in the React landscape.
Quick Links
Legal Stuff