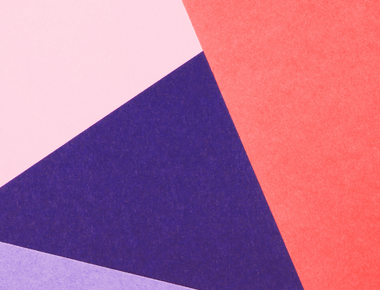
In recent years, gRPC has been making waves as a high-performance, open-source, and universal RPC framework. With ASP.NET Core’s native support for gRPC, developers can now easily integrate this powerful tool into their microservices architectures. In this blog post, we’ll demystify gRPC, highlight its advantages over traditional RESTful APIs, and walk you through a basic example using ASP.NET Core.
For the purpose of this tutorial, let’s create a simple gRPC service that lets users fetch and add messages.
a. Create a new ASP.NET Core gRPC service using:
dotnet new grpc -n MessageService
b. This will generate a Protos folder with a .proto file. Replace the contents of greet.proto with:
syntax = "proto3"; option csharp_namespace = "MessageService"; package Greet; service Message { rpc GetMessage (MessageRequest) returns (MessageReply); rpc AddMessage (AddMessageRequest) returns (MessageReply); } message MessageRequest { int32 id = 1; } message AddMessageRequest { string content = 1; } message MessageReply { string content = 1; }
Open ’Services/GreetService.cs’ and implement the service:
public class MessageService : Message.MessageBase { private static readonly Dictionary<int, string> Messages = new Dictionary<int, string> { [1] = "Hello, gRPC!", [2] = "Welcome to ASP.NET Core gRPC." }; public override Task<MessageReply> GetMessage(MessageRequest request, ServerCallContext context) { var content = Messages.GetValueOrDefault(request.Id, "Message not found."); return Task.FromResult(new MessageReply { Content = content }); } public override Task<MessageReply> AddMessage(AddMessageRequest request, ServerCallContext context) { int newId = Messages.Keys.Max() + 1; Messages[newId] = request.Content; return Task.FromResult(new MessageReply { Content = "Added Successfully!" }); } }
dotnet run
Your gRPC service is now running and ready to respond to GetMessage and AddMessage requests.
gRPC is undeniably a transformative protocol that bridges the performance and versatility gaps commonly associated with RESTful APIs. Its tight integration with Protocol Buffers ensures not only efficient serialization but also guarantees clear, strongly-typed contracts, making system integrations more predictable and safer. Furthermore, with the native support from ASP.NET Core, building and deploying a gRPC service has become even more seamless, allowing developers to harness the real-time capabilities and cross-language compatibility it offers. Whether you’re modernizing legacy systems or architecting new solutions, consider gRPC with ASP.NET Core as a formidable choice for building robust, performant, and scalable microservices.
Quick Links
Legal Stuff