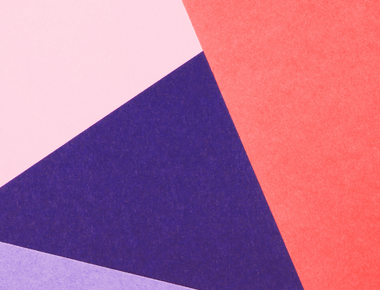
ASP.NET Core is designed from the start to support dependency injection. ASP.NET Core injects dependencies through a constructor or method using an IoC container.
The built-in container is represented by an IServiceProvider implementation that supports constructor injection. The types (classes) managed by built-in IoC container are called services.
There are two different types of services in ASP.NET Core:
To let the IoC container automatically instantiate our application services, we first need to register them with the IoC.
Consider this example of an ILog interface and its implementation class. It’s important to register modules with a built-in container before use, and we’ll look at how to do that next.
public interface ILog { void info(string str); } class MyConsoleLogger : ILog { public void info(string str) { Console.WriteLine(str); } }
ASP.NET Core lets you set up these services by registering them in the ConfigureServices method of the Startup class.
public void ConfigureServices(IServiceCollection services) { services.AddSingleton<ILog, MyConsoleLogger>(); services.AddSingleton(typeof(ILog), typeof(MyConsoleLogger)); }
With lifetime management built-in, the container automatically frees service instances based on their specified lifetimes.
The IoC container provided with the framework supports three types of lifetimes:
If a service type is included as a parameter in the constructor of an object, then the container automatically performs constructor injection.
For example, you can use the ILog type in any MVC controller. Consider the following example.
public class HomeController : Controller { ILog _log; public HomeController(ILog log) { _log = log; } public IActionResult Index() { _log.info("Executing /home/index"); return View(); } }
In this example, an IoC container will automatically pass a concrete instance of MyConsoleLogger to the constructor of HomeController. Registering an instance of ILog to the IoC container will automatically create or dispose it when required.
Sometime In a single action method, it may only be necessary to add dependency service type. Use the FromServices attribute in the method with parameter type service.
using Microsoft.AspNetCore.Mvc; public class HomeController : Controller { public HomeController() { } public IActionResult Index([FromServices] ILog log) { log.info("Index method executing"); return View(); } }
Dependency Injection (DI) stands as a pivotal feature in ASP.NET Core, enabling developers to maintain clean, modular, and loosely-coupled code. The framework provides a built-in IoC container that ensures seamless service registration and instantiation, whether they’re built into the framework or custom-made by the developers. With diverse service lifetimes – Singleton, Transient, and Scoped – the framework ensures optimal memory and resource management. Furthermore, ASP.NET Core goes beyond traditional constructor injection, offering the flexibility of action method injection for more granular control. By embracing these DI capabilities, developers can achieve a high degree of modularity and maintainability in their applications, ensuring robust, scalable, and efficient software solutions.
Quick Links
Legal Stuff