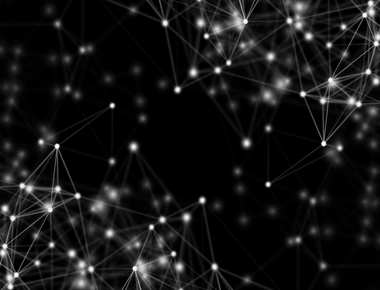
This section provides an overview of how to use Angular event hooks. Angular invokes the life cycle hooks on directives and components as it creates, changes, and destroys them. Using lifecycle hooks, we can further customize the behavior of our components during their creation, updating and destruction.
When starting the angular application, it creates and renders the root component. It then creates and renders its Children’s & their children. It forms a tree of components.
Once Angular loads the components, it begins the process of rendering the view. To do that, it needs to check the input properties, evaluate the data bindings and expressions in order to render projected content. Angular also removes the component from the DOM when it is no longer needed.
Angular lets us know when these events happen by using lifecycle hooks
The Angular life cycle hooks are simply callback function, which angular invokes when a specific event occurs during the component’s life cycle.
For example,
The following are complete list of life cycle hooks, which angular invokes during the component life cycle. Angular triggers these functions when a specific event happens.
Before we discuss lifecycle hooks, let us understand the change detection cycle.
The change detection mechanism is used by Angular to keep the component in sync with the template.
Consider the following code.
<div>Hello {{ name }}</div>
Angular updates the DOM as the value of a name
changes. And it does it instantly.
How does angular know when the value of name changes? It does this by running a change detection cycle on every event that may result in a change. It runs continuously, regardless of changes in input, DOM events, timer events like setTimeout() and setInterval() , http requests etc.
With the change detection cycle, Angular checks each and every bound property in the template, with that of the component class. If it detects any changes, it updates the DOM.
Angular raises life cycle hooks during the important stages of its change detection mechanism.
A component’s life cycle begins, when Angular creates the component class. The first method invoked is the class constructor.
Constructor is neither a life cycle hook nor it is specific to Angular. This is a Javascript feature. When you create a class, it invokes the constructor method.
Angular uses a constructor to inject dependencies.
None of the components input properties are yet available to use. Neither of its child components are constructed. Projected contents are also not available.
Hence there is not much you can do in this method. And it’s also recommended to avoid using this method.
When Angular instantiates the class, it kicks off a change detection cycle for the component.
Here is a code example demonstrating the use of these lifecycle hooks in an Angular component:
import { Component, OnInit, OnChanges, DoCheck, AfterContentInit, AfterContentChecked, AfterViewInit, AfterViewChecked, OnDestroy, SimpleChanges } from '@angular/core'; @Component({ selector: 'app-lifecycle-demo', template: ` <div>Content here...</div> `, }) export class LifecycleDemoComponent implements OnInit, OnChanges, DoCheck, AfterContentInit, AfterContentChecked, AfterViewInit, AfterViewChecked, OnDestroy { // Input property for demo purposes @Input() inputProp: any; constructor() { console.log('Constructor'); } ngOnChanges(changes: SimpleChanges): void { console.log('ngOnChanges - data-bound input property changed', changes); } ngOnInit(): void { console.log('ngOnInit - component initialization'); } ngDoCheck(): void { console.log('ngDoCheck - change detection run'); } ngAfterContentInit(): void { console.log('ngAfterContentInit - content (ng-content) initialized'); } ngAfterContentChecked(): void { console.log('ngAfterContentChecked - content (ng-content) checked'); } ngAfterViewInit(): void { console.log('ngAfterViewInit - component's view initialized'); } ngAfterViewChecked(): void { console.log('ngAfterViewChecked - component's view checked'); } ngOnDestroy(): void { console.log('ngOnDestroy - cleanup logic'); } }
You can use this component and see the logs in the browser console to understand the order in which these hooks are called.
Angular offers a comprehensive suite of lifecycle hooks that allow developers to closely monitor and intervene in different stages of a component’s life cycle. These lifecycle hooks provide us with a way to execute custom logic at specific moments, from initialization to teardown, ensuring that our applications are efficient and responsive. Whether it’s responding to data changes, optimizing performance, or cleaning up resources, these hooks play a crucial role in every Angular application. The example provided showcases the sequence and the usage of these hooks. As developers, leveraging these hooks effectively can make a significant difference in how our applications behave and perform.
Quick Links
Legal Stuff