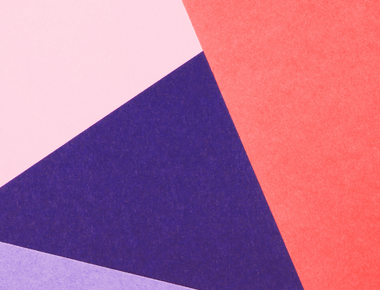
Concurrency is the backbone of modern software applications, enabling efficient multitasking and ensuring that applications remain responsive. In the Python world, ’asyncio’ has brought in a new era for concurrent programming. Let’s journey through the realms of ’asyncio’ and understand the magic of concurrency.
Before diving deep, it’s crucial to clarify the difference between concurrency and parallelism.
In Python, ’asyncio’ provides a framework for concurrency, primarily using co-routines, without the need for multi-threading or multi-processing.
Introduced in Python 3.3, ’asyncio’ is a library that offers asynchronous I/O, event loop, co-routines, and tasks. It’s designed to write concurrent code using the ’async’ and ’await’ syntax.
For example, a simple asynchronous function looks like:
import asyncio async def greet(): await asyncio.sleep(1) print("Hello, asyncio!") asyncio.run(greet())
In this example, the ’await’ keyword allows other parts of the event loop to run while ’asyncio.sleep’ simulates an I/O-bound operation.
At the heart of ’asyncio’ lies co-routines. A co-routine is a special function that can pause its execution (yield) and allow other co-routines to run.
Co-routines are started by calling them but return a co-routine object immediately without executing. To get them to execute, you schedule them in an event loop.
The event loop is the orchestrator of the show. It handles the execution of multiple I/O-bound operations without the need for threads or processes, managing tasks by pausing and resuming them in an optimized manner.
While ’asyncio’ is powerful, it’s essential to understand that not all problems benefit from asynchronous programming. CPU-bound tasks, for instance, might be better off using multi-threading or multi-processing. Furthermore, mixing asynchronous and synchronous code can be tricky and may lead to unexpected behaviors.
The evolution of software applications necessitates the adept management of multiple tasks, making concurrency an integral facet of modern development. In this context, Python’s ’asyncio’ emerges as a revolutionary tool, enabling seamless concurrency without delving into the complexities of multi-threading or multi-processing. Central to its power are co-routines, which, coupled with the event loop, offer an efficient means to handle I/O-bound operations. Benefits such as performance enhancement, resource efficiency, and maintainable code are undeniable with ’asyncio’. However, like all tools, it has its realm of optimal application. While it excels in scenarios like web scraping, server requests, or database queries, one must exercise caution and judgment when dealing with CPU-bound tasks or when intertwining synchronous with asynchronous code. In sum, while ’asyncio’ is a formidable tool in a developer’s arsenal, its effectiveness is ultimately determined by its judicious application.
Quick Links
Legal Stuff